Most apps have some sort of authentication. For this post, we will see how this flow works using Google’s One Tap sign-in, Firebase and Amity.
The tech stack we will be using is:
- Kotlin script (KTS) for our Gradle
- Jetpack Compose for our UI
- MVVM architecture
- Hilt for dependency injection
- Amity’s Social SDK
- Google’s OneTap authentication
- Firebase authentication
The flow is as follows: first, we’ll check if Amity’s session is valid; if it is we’ll continue to our main flow, if not we will redirect our users to our log-in screen. There we will first try to log our users in using Google. If they’ve logged in to our app before this will succeed and then we can log in using Firebase. If not, we first need to sign up our users to our app, and then continue with Firebase.
Configuration
Alright, let’s begin! First, to configure our project we’ll use the official guide. Since we don’t use Groovy, the dependencies are added as shown below.
*While you should use the provided <span id="greylight" class="greylight">libs.versions</span> file (versions catalog), for the sake of code readability in this post we’ll add the versions like this.
Since we’re here, we’ll also add the Amity and Firebase dependencies that we’ll need later. Adding Firebase dependencies in the template, unfortunately, cannot be done yet via the Android Studio’s Assistant without throwing exceptions so we’ll add those manually.
Our last step is to configure our Firebase console. Per the official documentation:
To use an authentication provider, you need to enable it in the Firebase console. Go to the Sign-in Method page in the Firebase Authentication section to enable Email/Password sign-in and any other identity providers you want for your app.
We’ll of course enable the Google sign-in. Then we’ll navigate to the project settings and add our SHA certificate fingerprint.
Now we’re ready to start adding our login code! We first create the files we’ll need: <span id="greylight" class="greylight">MainNavigation</span> , <span id="greylight" class="greylight">MainViewModel</span> , <span id="greylight" class="greylight">LoginScreen</span> , <span id="greylight" class="greylight">LoginViewModel</span> , <span id="greylight" class="greylight">AuthRepository</span> .
Authentication status observation
Our first Composable to be called is the <span id="greylight" class="greylight">MainNavigation</span>. To constantly check Amity’s session validity this is where we’ll monitor its state. Since we want our app’s state to reflect the session’s state we will map it to a <span id="greylight" class="greylight">StateFlow</span> .
StateFlow is a state-holder observable flow that emits the current and new state updates to its collectors. The current state value can also be read through its value property. In Android, StateFlow is a great fit for classes that need to maintain an observable mutable state.
We initiate and get the <span id="greylight" class="greylight">SessionState</span> in the <span id="greylight" class="greylight">AuthRepository</span> , then in our <span id="greylight" class="greylight">MainViewModel</span> we convert our <span id="greylight" class="greylight">flow</span> to a <span id="greylight" class="greylight">StateFlow</span> , and finally in our <span id="greylight" class="greylight">MainNavigation</span> we observe it.
Cool, now we can observe our users' state! 🎉🎉🎉
Google OneTap sign-in
Our users now see our ✨shiny✨ login page which really for now is just the following
Not that shiny after all 😛
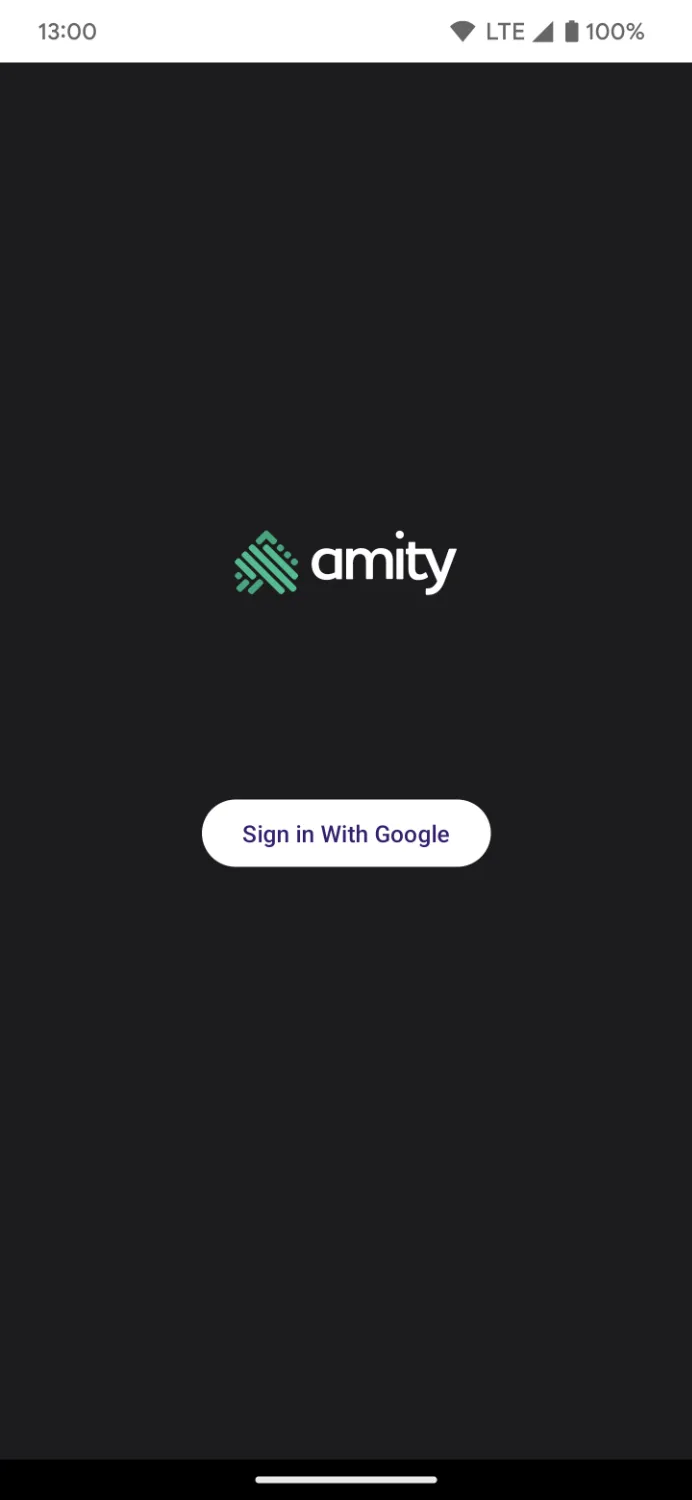
To begin, we need our sign-in call in our <span id="greylight" class="greylight">AuthRepository</span> . *
* :
We’re using an interface to encapsulate our <span id="greylight" class="greylight">AuthRepository</span> ‘s functions. This will be specifically useful for creating a mock <span id="greylight" class="greylight">AuthRepository</span> for our tests in the future.
Our <span id="greylight" class="greylight">signInRequest</span> , <span id="greylight" class="greylight">SignUpRequest</span> as well as the Firebase and the Google clients are provided to our <span id="greylight" class="greylight">AuthRepository</span> implementation during the dependency injection as shown below.
Back to our sign-in flow! As mentioned above, if it’s the first time our user’s trying to sign-in with this account, this will throw an exception. To avoid showing false error messages to the user, when we get an exception in our sign-in method, we’ll try to sign up. If that’s successful we move on, if not we then handle the error.
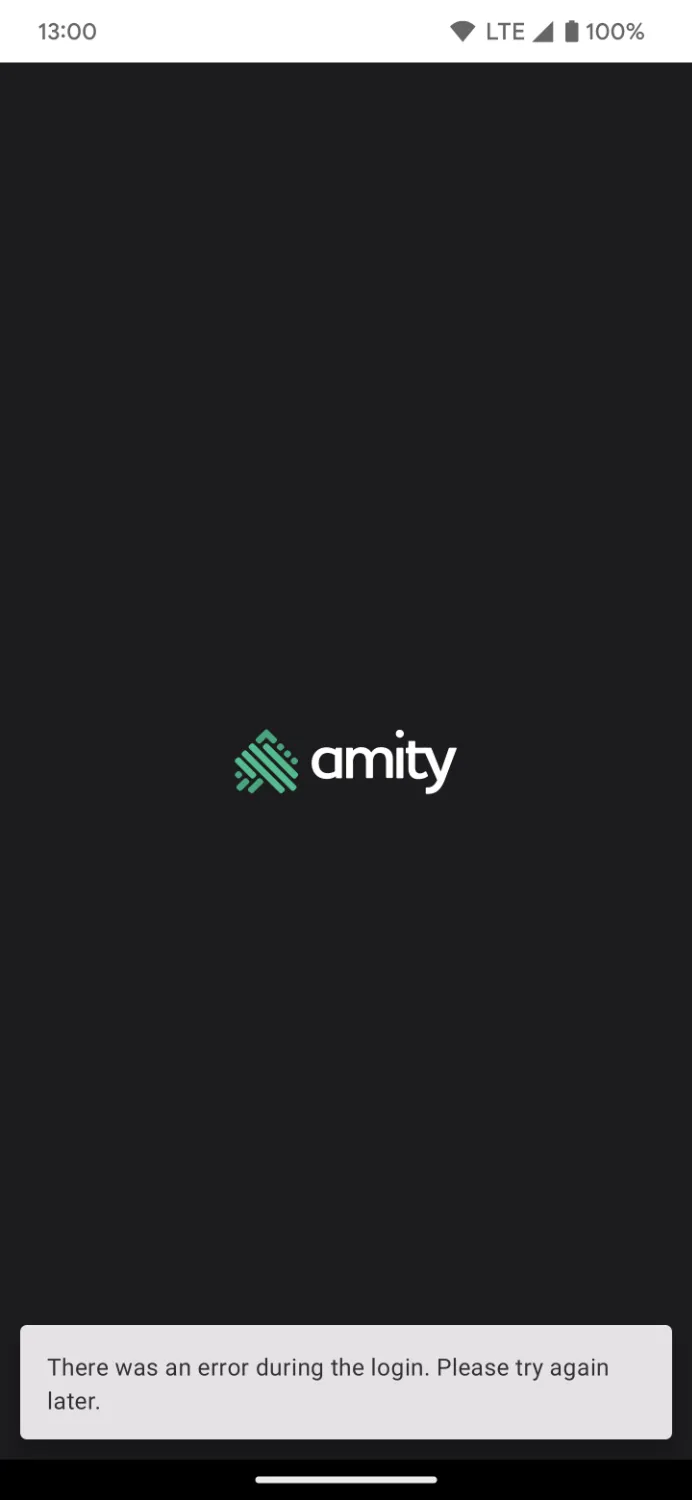
If we were using Views instead of Compose, we would handle the result of the intent in our <span id="greylight" class="greylight">onActivityForResult</span> method. Instead, we will use the <span id="greylight" class="greylight">ManagedActivityResultLauncher</span> in our <span id="greylight" class="greylight">LoginScreen</span> . If our launcher comes back with a positive result, we’ll then get our user’s ID and move on with the Firebase sign-in.
Firebase and Amity sign-in
Oof, almost done!!!
Back to our <span id="greylight" class="greylight">AuthRepository</span> first, we add our calls for Firebase and Amity.
If the user is new we’ll also add it to Firestore as it’s needed for our chat app, but this is not mandatory. Then in our LoginViewModel we call our suspend functions:
and replace our TO-DO with this call in our launcher.
Navigating away our login
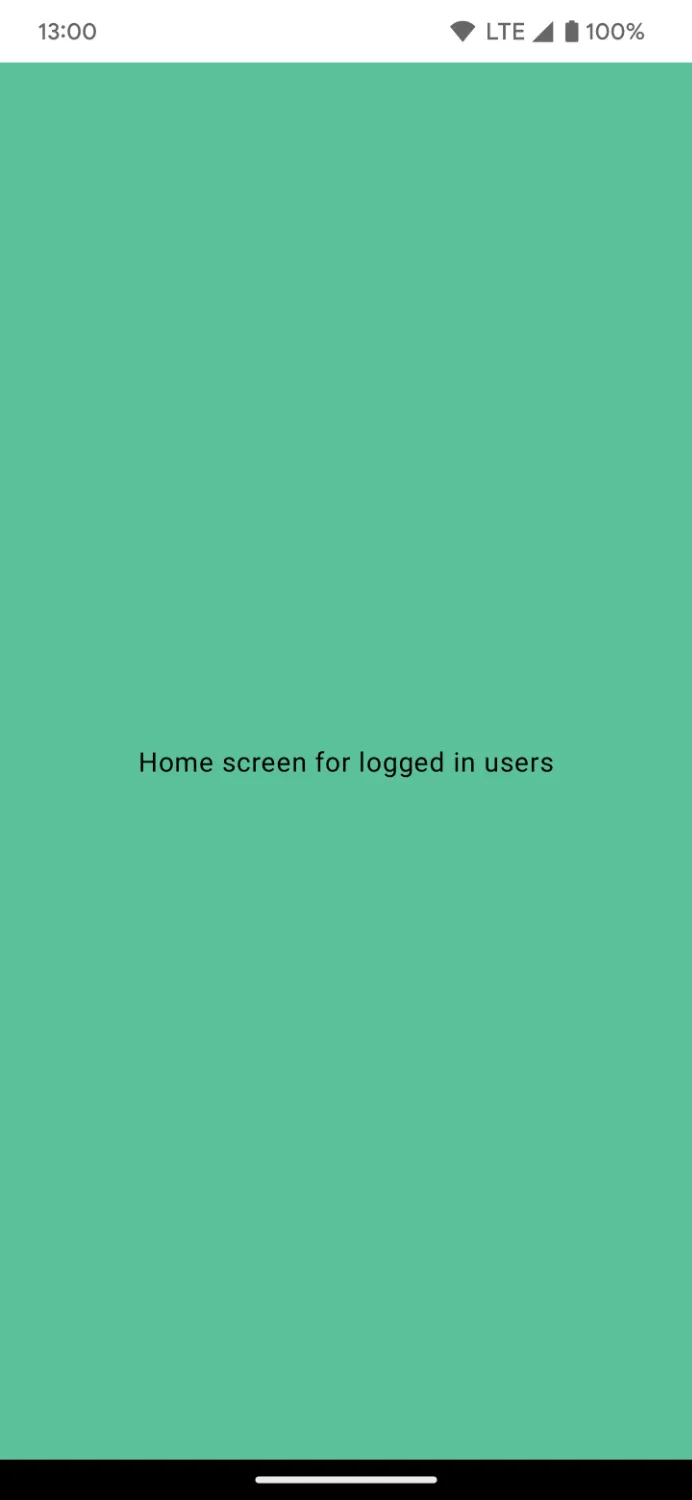
…or else observing our sign-in process state. Our last step, I promise!
We’ll start from our <span id="greylight" class="greylight">AuthRepository</span> again, and add our final call.
Since this is a callback, it means that every time the <span id="greylight" class="greylight">auth.currentUser</span> value changes, we will get notified. Then in our <span id="greylight" class="greylight">LoginViewModel</span> we map it to a UI state, and of course observe it to our <span id="greylight" class="greylight">LoginScreen</span> .
Access the full code for this tutorial here.